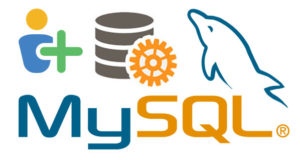
Summary: in this tutorial, you will learn how to use the MySQL CREATE USER
statement to create a new user in the MySQL database server.
Typically, MySQL creates the root user account during installation. This root user account has full privileges over the MySQL database server, giving it complete control over all databases, tables, users, and more.
It’s a good practice to use the root account for administrative functions exclusively. For other tasks, create a new user account and grant the necessary privileges.
This tutorial focuses on using the CREATE USER
statement to create a new user in the MySQL database.
Introduction to MySQL CREATE USER statement
To create a new user in the MySQL database, you use the CREATE USER
statement.
Here’s the basic syntax of the CREATE USER
statement:
CREATE USER [IF NOT EXISTS] account_name
IDENTIFIED BY 'password';
Code language: SQL (Structured Query Language) (sql)
In this syntax:
First, specify the account name after the CREATE USER
keywords. The account name consists of two parts: username
and hostname
, separated by the @
sign:
username@hostname
Code language: SQL (Structured Query Language) (sql)
The username
is the name of the user while the hostname
is the name of the host from which the user connects to the MySQL Server.
The hostname
part of the account name is optional. If you omit the hostname, the user can connect from any host.
An account name without a hostname is equivalent to the following:
username@%
Code language: SQL (Structured Query Language) (sql)
If the username
and hostname
contains special characters, such as spaces or hyphens, you need to enclose the username and hostname separately in quotes, like this:
'bob-cat'@'hostname'
Code language: SQL (Structured Query Language) (sql)
In addition to the single quote ('
), you can use backticks ( `
) or double quotation mark ("
).
Second, specify the password for the user after the IDENTIFIED BY
keywords.
The IF NOT EXISTS
option creates a new user only if it does not already exist. If the user exists, the statement issues a warning.
Note that the CREATE USER
statement creates a new user without any privileges. To grant privileges to the user, you use the GRANT
statement.
MySQL CREATE USER example
First, establish a connection to the MySQL Server using the mysql client tool with the root user account:
mysql -u root -p
Code language: SQL (Structured Query Language) (sql)
Enter the password for the root
account and press Enter
:
Enter password: ********
Code language: SQL (Structured Query Language) (sql)
Second, show the users on the current MySQL Server:
SELECT
user
FROM
mysql.user;
Code language: SQL (Structured Query Language) (sql)
Here is the output:
+------------------+
| user |
+------------------+
| mysql.infoschema |
| mysql.saession |
| mysql.sys |
| root |
+------------------+
Third, create a new user named bob
:
create user bob@localhost
identified by 'Secure1pass!';
Code language: SQL (Structured Query Language) (sql)
Fourth, show all users again:
select user from mysql.user;
Code language: SQL (Structured Query Language) (sql)
The output will be:
+------------------+
| user |
+------------------+
| bob |
| mysql.infoschema |
| mysql.session |
| mysql.sys |
| root |
+------------------+
5 rows in set (0.00 sec)
Code language: JavaScript (javascript)
The output indicates that the user with the name bob
has been created successfully.
Fifth, open a second session and log in to the MySQL database server with the user bob
:
mysql -u bob -p
Code language: SQL (Structured Query Language) (sql)
Input the password for bob
and press Enter
:
Enter password: ********
Code language: SQL (Structured Query Language) (sql)
Sixth, show the databases that bob
has access:
show databases;
Code language: SQL (Structured Query Language) (sql)
Here is the list of databases that bob
can access:
+--------------------+
| Database |
+--------------------+
| information_schema |
| performance_schema |
+--------------------+
2 rows in set (0.01 sec)
Code language: JavaScript (javascript)
Seventh, go to the session of the user root
and create a new database called bobdb
:
create database bobdb;
Code language: SQL (Structured Query Language) (sql)
Eight, select the database bobdb
:
use bobdb;
Code language: SQL (Structured Query Language) (sql)
Ninth, create a new table called todos
:
create table todos(
id int auto_increment primary key,
title varchar(255) not null,
completed bool default false
);
Code language: SQL (Structured Query Language) (sql)
Tenth, grant all privileges to the bobdb
to bob
:
grant all privileges on bobdb.* to bob@localhost;
Code language: SQL (Structured Query Language) (sql)
Note that you will learn how to grant privileges to a user in the GRANT
tutorial.
Eleventh, go to bob’s session and display all the databases:
show databases;
Code language: SQL (Structured Query Language) (sql)
Now, bob
can see the bobdb
:
+--------------------+
| Database |
+--------------------+
| bobdb |
| information_schema |
| performance_schema |
+--------------------+
3 rows in set (0.00 sec)
Code language: JavaScript (javascript)
Twelfth, select the database bobdb
:
use bobdb;
Code language: SQL (Structured Query Language) (sql)
Thirteenth, show the tables from the bobdb
database:
show tables;
Code language: SQL (Structured Query Language) (sql)
The user bob
can see the lists
table:
+-----------------+
| Tables_in_bobdb |
+-----------------+
| todos |
+-----------------+
1 row in set (0.00 sec)
Code language: JavaScript (javascript)
Fourteenth, insert a row into the todos
table:
insert into todos(title)
values('Learn MySQL');
Code language: SQL (Structured Query Language) (sql)
Fifteenth, query data from the lists
table:
select * from todos;
Code language: SQL (Structured Query Language) (sql)
This is the output:
+----+-------------+-----------+
| id | title | completed |
+----+-------------+-----------+
| 1 | Learn MySQL | 0 |
+----+-------------+-----------+
1 row in set (0.00 sec)
Code language: JavaScript (javascript)
So the user bob
can do everything with the bobdb
database.
Finally, disconnect from the MySQL Server from both sessions:
exit
Code language: SQL (Structured Query Language) (sql)
Summary
- Use the
CREATE USER
statement to create a new user in the MySQL Database Server.